Clojurescript, re-frame and P5
I’m trying out creating generative art using clojurescript and quil (a clojurescript implementation of P5).
Routing and directory structure
The main issue to be solved was to get routing working, so that I don’t have to go into the source code to switch between visuals.
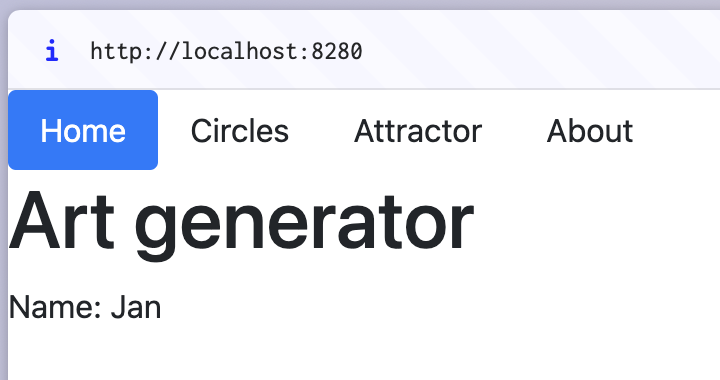
In the previous post of visualisation in clojurescript I created rect
and circle
in the views.cljs
file. That’s not how it should be done. The views.cljs
file should contain the different pages instead.
|
|
To add a tab that shows circles (using quil), we create a new file generators/circles.cljs
:
|
|
In the views.cljs
file we add circles.cljs
to the :require
and create a new view:
|
|
Unfortunately, this does not always work. The problem seems to be that the div
with id sketch
is not mounted yet before the image is created and is attached to this (non-existing) div.
Workaround
A possible workaround is to - instead of generating the image immediately - ask for the user to click a button first. When clicked, the image is created and mounted in that div.
|
|
The result before clicking:
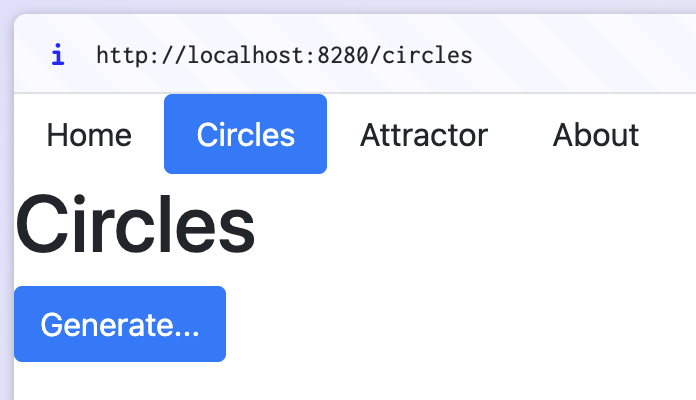
After clicking:
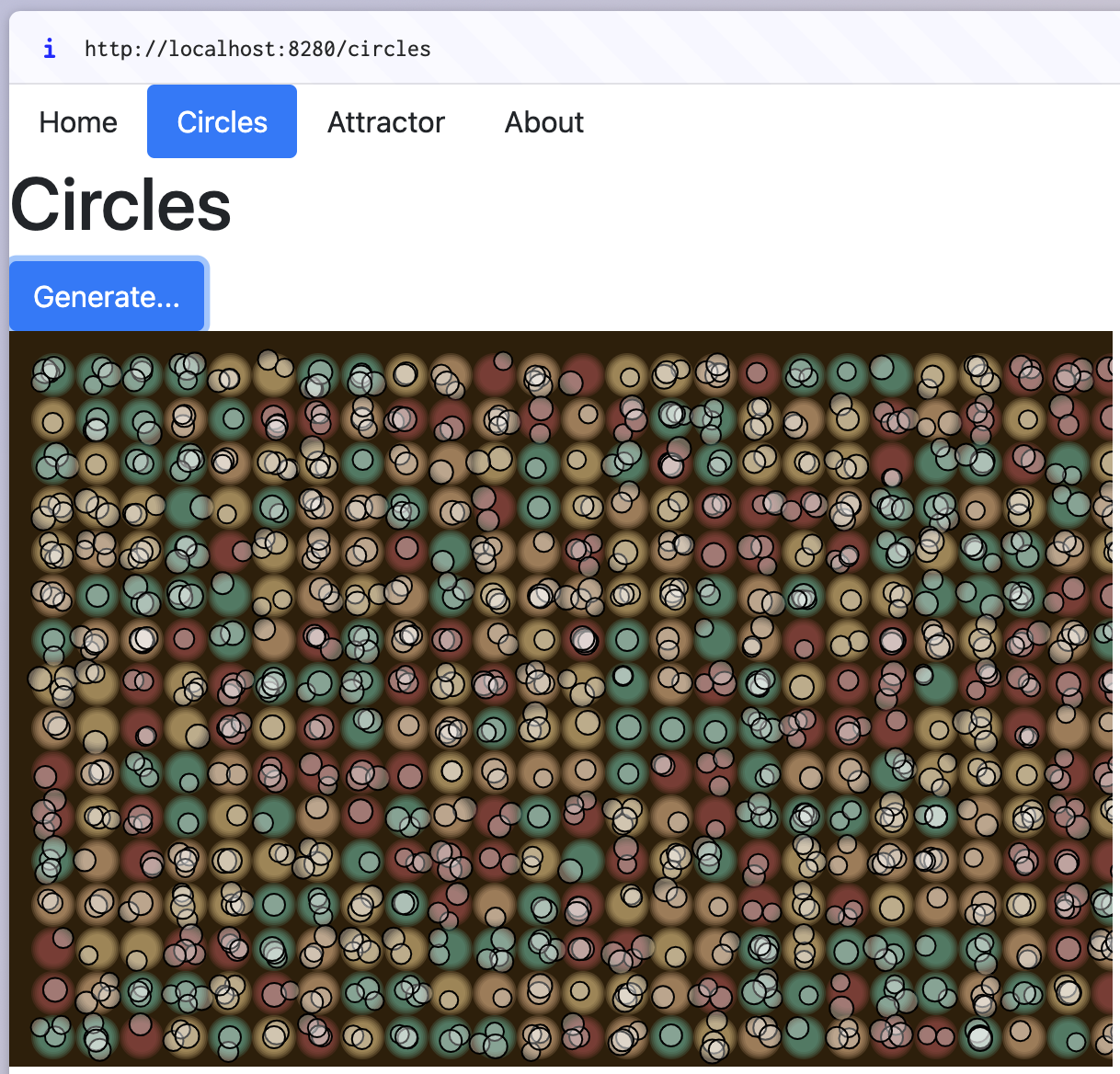